728x90
드림코딩 엘리님 강의 복습
// JavaScript is very flexible
// flexible === dangerous
// added ECMAScript 5
//use 'use strict' for Vanilla JS
'use strict';
자바스크립트는 막쓰기가 가능해서 오류가 일어나기 쉬움
파일 제일 앞에 'use strict' 를 써줌으로써 너무 막 쓰는 거 방지 가능
//2. Variable
//let (added in ES6)
// mutable type == 변하기 쉬운 타입
//global은 최소한이 좋음 클래스나 함수 if나for문에 사용
let globalName = 'global'
{
let name = 'hayeong';
console.log(name);
name = 'hello';
console.log(name);
console.log(globalName);
}
console.log(globalName);
//var 쓰면 안됨
//var hoisting (move declaration from bottom to top)
//어디에 선언했느냐에 상관없이 항상 제일 위로 선언을 끌어올려주는 것
//has no block scope!
{
age = 4;
var age;
}
console.log(age);
-선언 먼저 안해도 변수할당가능;;
//3.Constants
//favor immutable data type always for a few reasons;
//-security
//-thread safety
//-reduce human mistakes
//let과 다르게 한 번 할당하면 바뀌지않음.
const daysInWeek = 7;
const maxNumber = 5;
//4. Variable types
//primitive, single itme: number, string, boolean, null, undefined, symbol
//object, box container
//function, first-class function
const count = 17; // integer
const size = 17.1; // decimal number
console.log(`value: ${count}, type: ${typeof count}`);
console.log(`value: ${globalName}, type: ${typeof gobalName}`);
//number - special numeric values: infinity, -infinity, NaN
const infinity = 1/0;
const negativeInfinity = -1/0;
const nAn = 'not a number' / 2;
console.log(infinity);
console.log(negativeInfinity);
console.log(nAn);
//bigInt (fairly new, don't use it yet) - 엘리님이 강의하실때는 이 데이터 타입이 크롬에만 적용 가능했는데 지금은 인터넷 익스플로러빼고 다 가능한것으로 확인.
const bigInt = 1232424325235423n; // over (-2**53) ~ 2*53
console.log(`value: ${bigInt}, type: ${typeof bigInt} `)
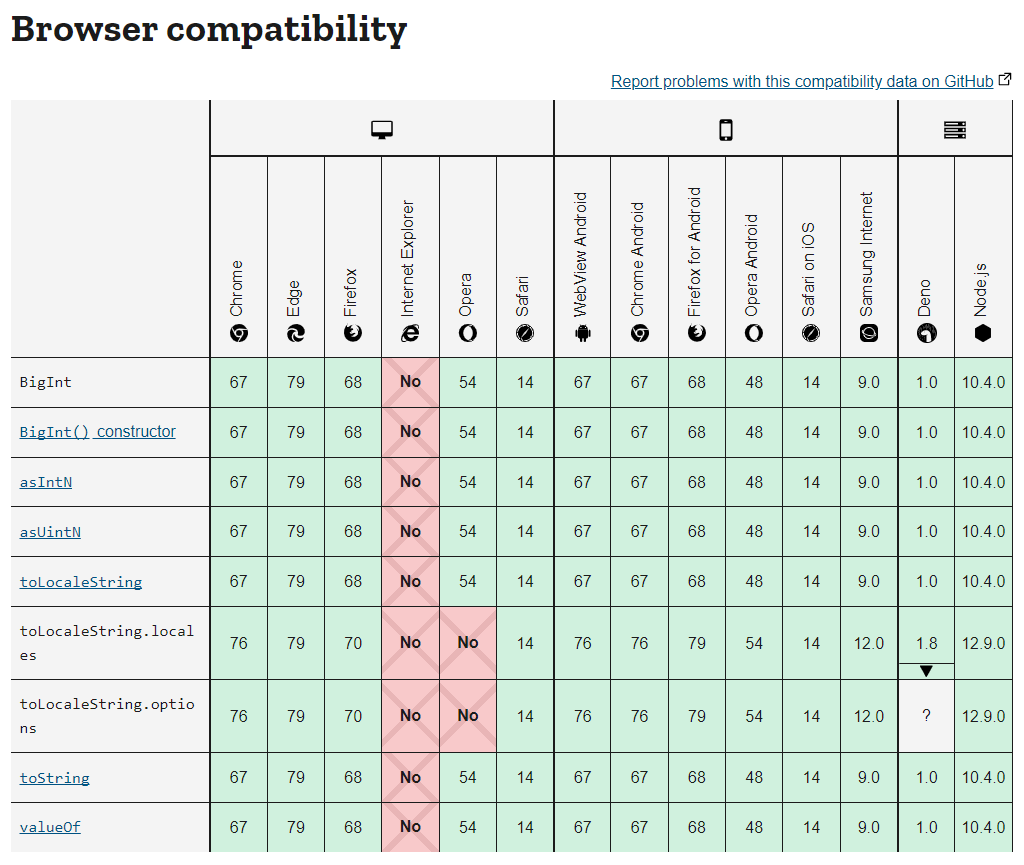
// string
const char = 'c';
const brendan = 'brandan';
const greeting = 'hello' + brendan;
console.log(`value: ${greeting}, type: ${typeof greeting}`);
const helloBob = `hi ${brendan}!`; // template literals (string)
console.log(`value: ${helloBob}, type: ${typeof helloBob}`);
console.log('value: ' + helloBob + ' type: ' + typeof helloBob);
//boolean
//false: 0, null, undefined, NaN, ''
//true: any other value
const canRead = true;
const test = 3 < 1;
console.log(`value: ${canRead}, type: ${typeof canRead}`);
console.log(`value: ${test}, type: ${typeof test}`);
//null
let nothing = null;
console.log(`value: ${nothing}, type: ${typeof nothing}`)
//undefined
let x;
console.log(`value: ${x}, type: ${typeof x}`)
//symbol, create unique identificatifier for objects
const symbol1 = Symbol('id');
const symbol2 = Symbol('id');
console.log(symbol1 === symbol2);
const gsymbol1 = Symbol.for('id');
const gsymbol2 = Symbol.for('id');
console.log(gsymbol1 === symbol2); // true
console.log(`value: ${symbol1.description}, type: ${typeof symbol1.description}`);
// object, real-life objects, data structure
const ellie = { name: 'ellie', age: 20};
ellie.age = 21;
console.log(ellie);
ellie라는 변수안에 할당 된 변수들은 변경가능.
// 5. Dynamic typing: dynamically typed language
// 변수를 선언할때 어떤 타입인지 선언하지 않고 프로그램이 동작할때 할당된 값에따라 타입이 변경 됨.
// <> Statically typed language = C, JAVA - 변수를 선언할 때 어떤 타입인지 결정해서 타입을 같이 선언.
//다이나믹 - 대규모에는 안좋음
let text = 'hello';
console.log(text.charAt(0)); // h
console.log(`value: ${text}, type: ${typeof text}`);
text = 1;
console.log(`value: ${text}, type: ${typeof text}`);
text = '7' + 5;
console.log(`value: ${text}, type: ${typeof text}`);
text = '8' / '2';
console.log(`value: ${text}, type: ${typeof text}`);
console.log(text.charAt(0));//에러! 다이나믹 타이핑의 단점 - 나중에 타입이 바뀔 수 있음. - 대안으로 타입스크립트나옴
돔요소 - html 문서의 tag들 js로 움직일 수 있는 것들
조금밖에 안했지만 넘 재밌음
엘리님이 재밌게 잘 가르쳐주셔서 그런 듯
ㅠㅠ 감사합니다
728x90
'study > js' 카테고리의 다른 글
JavaScript Array 정리! (feat. 드림코딩 앨리님) (0) | 2022.01.10 |
---|---|
JavaScript Object 정리 (feat. DreamCoding Ellie~) (0) | 2022.01.09 |
자바스크립트 클래스와 오브젝트! (feat. 드림코딩 by 엘리님) (0) | 2022.01.08 |
자바스크립트 함수 개념 정리(feat. DreamCoding Ellie) (0) | 2022.01.07 |
자바스크립트 연산자 반복문 11가지(feat. 드림코딩 앨리님♡) (0) | 2022.01.07 |
댓글